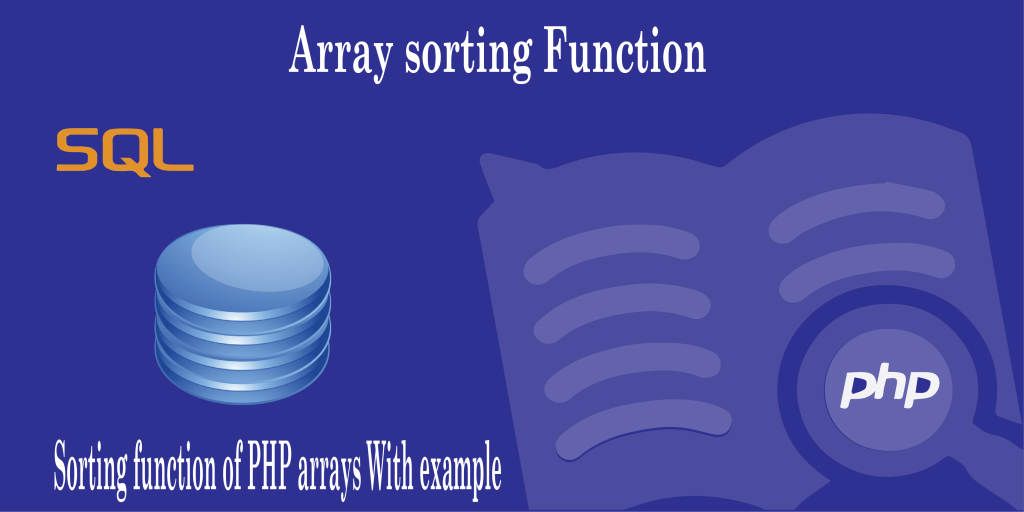
What is sorting?
Sorting is a way in PHP to order data in an alphabetical, numerical, and increasing or decreasing order fashion according to some linear relationship among the data items. Sorting greatly builds the efficiency of searching.
There are six Sorting functions for Arrays in PHP
- sort() – Ascending order in sorts arrays
- rsort() – Descending order in sorts arrays
- asort() – Ascending order in sorts associative arrays, according to the value
- ksort() – Ascending order in sorts associative arrays, according to the key
- arsort() –Descending order in sorts associative arrays, according to the value
- krsort() –Descending order in sorts associative arrays, according to the key
Ascending Order in Sort Array – sort()
The following function sorts the elements of a numerical array in ascending numerical order:
INPUT :
<?php
$numbers = array(40, 61, 2, 22, 13);
sort($numbers);
$arrlength = count($numbers);
for($x = 0; $x < $arrlength; $x++) {
echo $numbers[$x];
echo "<br>";
}
?>
OUTPUT :
2
13
22
40
61
Descending Order in Sort Array – rsort()
The following function sorts the elements of a numerical array in descending numerical order:
INPUT :
<?php
$numbers = array(40, 61, 2, 22, 13);
rsort($numbers);
$arrlength = count($numbers);
for($x = 0; $x < $arrlength; $x++) {
echo $numbers[$x];
echo "<br>";
}
?>
OUTPUT :
61
40
22
13
2
Ascending Order in Sort Array, According to Value – asort()
The following function sorts an associative array in ascending order, according to the value:
INPUT :
<?php
$age = array("Mayur"=>"23", "Pritakh"=>"47", "Shailesh"=>"41");
asort($age);
foreach($age as $x => $x_value) {
echo "Key=" . $x . ", Value=" . $x_value;
echo "<br>";
}
?>
OUTPUT :
Key=Mayur, Value=23
Key=Pritakh, Value=41
Key=Shailesh, Value=47
Ascending Order in Sort Array, According to Key – ksort()
The following function sorts an associative array in ascending order, according to the key:
INPUT :
<?php
$age = array("Mayur"=>"23", "Pritakh"=>"47", "Shailesh"=>"41");
ksort($age);
foreach($age as $x => $x_value) {
echo "Key=" . $x . ", Value=" . $x_value;
echo "<br>";
}
?>
OUTPUT :
Key=Mayur, Value=23
Key=Pritakh, Value=41
Key=Shailesh, Value=47
Descending Order in Sort Array, According to Value – arsort()
The following function sorts an associative array in descending order, according to the value.
INPUT :
<?php
$age = array("Mayur"=>"23", "Pritakh"=>"47", "Shailesh"=>"41");
arsort($age);
foreach($age as $x => $x_value) {
echo "Key=" . $x . ", Value=" . $x_value;
echo "<br>";
}
?>
OUTPUT :
Key=Mayur, Value=47
Key=Pritakh, Value=41
Key=Shailesh, Value=23
Descending Order in Sort Array, According to Key – krsort()
The following function sorts an associative array in descending order, according to the key.
INPUT :
<?php
$age = array("Mayur"=>"23", "Pritakh"=>"47", "Shailesh"=>"41");
krsort($age);
foreach($age as $x => $x_value) {
echo "Key=" . $x . ", Value=" . $x_value;
echo "<br>";
}
?>
OUTPUT :
Key=Mayur, Value=47
Key=Pritakh, Value=41
Key=Shailesh, Value=23