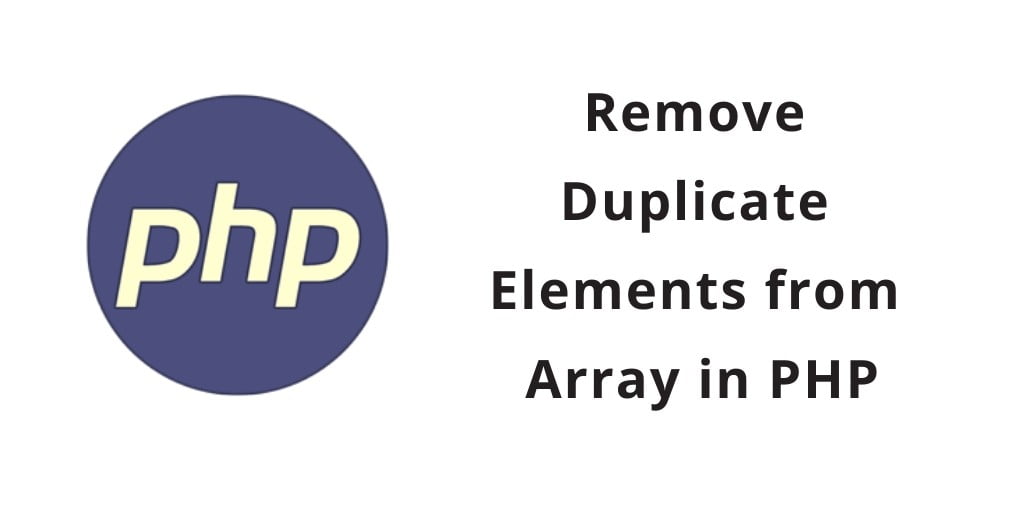
In this query, we will discuss How to remove duplicate elements from an array in PHP. We can get the unique elements by using array_unique() function. This function will remove the duplicate values from the array.
Parameters: This function accepts two parameters that are discussed below:
- $array: This parameter is mandatory to be supplied and it specifies the input array from which we want to remove duplicates.
- $sort_flags: It is an optional parameter. This parameter may be used to modify the sorting behavior using these values:
- SORT_REGULAR: This is the default value of the parameter $sort_flags. This value tells the function to compare items normally (don’t change types).
- SORT_NUMERIC: This value tells the function to compare items numerically.
- SORT_STRING: This value tells the function to compare items as strings.
- SORT_LOCALE_STRING: This value tells the function to compare items as strings, based on the current locale.
- Return Value: The array_unique() function returns the filtered value after removing all duplicates from the array.
Example: PHP program to remove duplicate values from the array.
<?php
// Input Array
$a = array("circle", "square", "circle", "triangle");
// Array after removing duplicates
print_r(array_unique($a));
?>
Output
Array
(
[0] => circle
[1] => square
[3] => triangle
)
Example: PHP program to remove duplicate elements from an associative array.
<?php
// Input array
$arr = array(
"a" => "BH",
"b" => "CK",
"c" => "CK",
"d" => "AND"
);
// Array after removing duplicates
print_r(array_unique($arr));
?>
Output
Array
(
[a] => BH
[b] => CK
[d] => AND
)