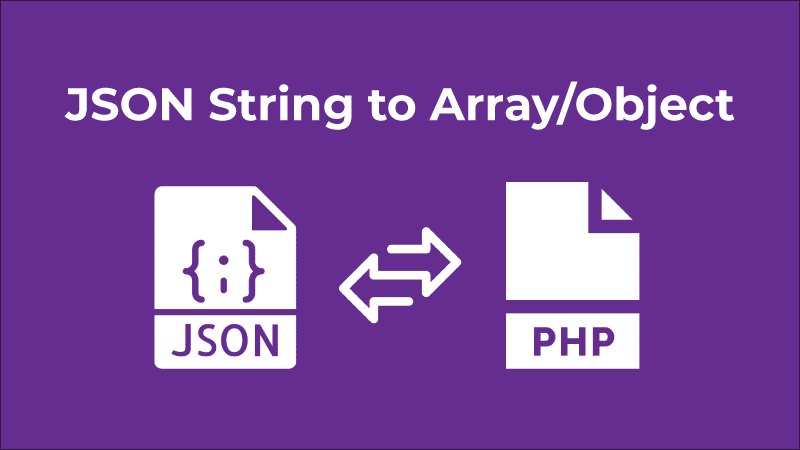
In this Query, we will see How to convert a JSON string to an array in PHP, & will see its implementation through examples.
There are three types of arrays in PHP that are listed below:
Indexed Array: It is an array with a numeric key. It is basically an array wherein each of the keys is associated with its own specific value.
Associative Array: It is used to store key-value pairs.
Multidimensional Array: It is a type of array which stores another array at each index instead of a single element. In other words, define multi-dimensional arrays as arrays of arrays. For this purpose, we will use an associative array that uses a key-value type structure for storing data. These keys will be a string or an integer which will be used as an index to search the corresponding value in the array.
The json_encode() function is used to convert the value of the array into JSON. This function is added in from PHP5. Also, you can make more nesting of arrays as per your requirement. You can also create an array of array of objects with this function. As in JSON, everything is stored as a key-value pair we will convert these key-value pairs of PHP arrays to JSON which can be used to send the response from the REST API server.
Example: The below example is to convert an array into JSON.
<?php
$arr = array (
// Every array will be converted
// to an object
array(
"name" => "Pranav Sharma",
"age" => "21"
),
array(
"name" => "Rohit Mehta",
"age" => "20"
),
array(
"name" => "Arpita Agarwal",
"age" => "21"
)
);
// Function to convert array into JSON
echo json_encode($arr);
?>
Output:
[{“name”:”Pranav Sharma”,”age”:”21″},
{“name”:”Rohit Mehta”,”age”:”20″},
{“name”:”Arpita Agarwal”,”age”:”21″}]
Example: This example depicts the conversion of the 2D associative array into JSON.
<?php
$arr = array (
"first"=>array(
"id"=>1,
"product_name"=>"Circuitboard",
"cost"=>200
),
"second"=>array(
"id"=>2,
"product_name"=>"Tiffin",
"cost"=>98
),
"third"=>array(
"id"=>3,
"product_name"=>"Air Conditioner",
"cost"=>8000
)
);
// Function to convert array into JSON
echo json_encode($arr);
?>
Output:
{“first”:{“id”:1,”product_name”:”Circuitboard”,”cost”:200},
“second”:{“id”:2,”product_name”:”Tiffin”,”cost”:98},
“third”:{“id”:3,”product_name”:”Air Conditioner”,”cost”:8000}}